(C#) I'm trying to convert bytes (from an image) that are not ASCII-printable into a string.
Meaning..
System.Text.ASCIIEncoding enc = new System.Text.ASCIIEncoding();
str = enc.GetString(myBytes);
..does not work, it will show ???? characters.
Any help appreciated.
C# Non-ASCII-Printable Bytes To String
C# Non-ASCII-Printable Bytes To String


[ AI Revision ][ Sewer ][ Boat Mod ][ Archaic ]
Remapped: Mod Archive and Forums.
- LuxuriousMeat
- Posts: 824
- Joined: Thu Nov 03, 2005 6:43 pm
- Location: zzzzzzzzzzzzzzzz
- Contact:
Re: C# Non-ASCII-Printable Bytes To String
Uh, are you trying to like show the hexadecimal value of the bytes?Zone 117 wrote:(C#) I'm trying to convert bytes (from an image) that are not ASCII-printable into a string.
Meaning..
System.Text.ASCIIEncoding enc = new System.Text.ASCIIEncoding();
str = enc.GetString(myBytes);
..does not work, it will show ???? characters.
Any help appreciated.
If so use this:
Code: Select all
str = BitConverter.GetString(myBytes);
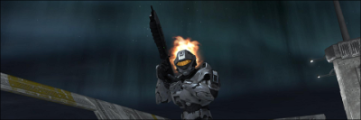
Re: C# Non-ASCII-Printable Bytes To String
I started trying to use the BitConverter a little bit ago. As far as I can see, it converts the bytes to hex correctly. However when I convert the hex back into bytes, half the file is empty.
What method would you use to convert them back into bytes?
Thanks.
What method would you use to convert them back into bytes?
Thanks.


[ AI Revision ][ Sewer ][ Boat Mod ][ Archaic ]
Remapped: Mod Archive and Forums.
- LuxuriousMeat
- Posts: 824
- Joined: Thu Nov 03, 2005 6:43 pm
- Location: zzzzzzzzzzzzzzzz
- Contact:
Re: C# Non-ASCII-Printable Bytes To String
Here's one I use:Zone 117 wrote:I started trying to use the BitConverter a little bit ago. As far as I can see, it converts the bytes to hex correctly. However when I convert the hex back into bytes, half the file is empty.
What method would you use to convert them back into bytes?
Thanks.
Code: Select all
byte[] TextStringToBytes(string str)
{
string[] parts = str.Split(new char[] { '-' });
if (parts.Length < 1) return new byte[0];
byte[] bytes = new byte[parts.Length];
for (int x = 0; x < parts.Length; x++)
if (!byte.TryParse(parts[x], out bytes[x])) return new byte[0];
return bytes;
}
Code: Select all
string str = "00-02-03-04";
byte[] b = TextStringToBytes(str);
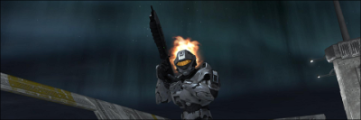