Some C# Questions
- WaywornMmmmm
- Posts: 1341
- Joined: Sat Nov 05, 2005 5:17 am
- Location: U.S.A
![]() |
Some C# Questions
I have some more questions.
Is there a way to have c# to check if a variable is a perfect square?
Also, is there a way to add a new form to my project which will be an MDIContainer for the original and any new forms I may add?
Also, this has been edited so some responses don't flow with these questions.
Thanks for any help.
Is there a way to have c# to check if a variable is a perfect square?
Also, is there a way to add a new form to my project which will be an MDIContainer for the original and any new forms I may add?
Also, this has been edited so some responses don't flow with these questions.
Thanks for any help.
Last edited by WaywornMmmmm on Mon Jul 16, 2007 10:18 am, edited 1 time in total.
i've never seen a lick of C# code in my life, but in C++ it would look something like this:
Code: Select all
#include <iostream> /*for input/output stream support. we need this to use cin for the input.*/
int main()
{
int input; //the int variable the user's input will be stored in.
std::cout << "\nenter the numbers: "; //the output to tell the user what to do
std::cin >> input; //cin takes what they type in and stores it in input
/*note: when cin encounters a space, it stops reading from the stream. for a single number, that shouldn't be a problem, but it causes issues when you get into strings...*/
return(0); //end the program, and return 0 (everything's fine)
}
ASPARTAME: in your diet soda and artificial sweeteners. also, it's obviously completely safe. it's not like it will cause tumors or anything. >.>
always remember: guilty until proven innocent
always remember: guilty until proven innocent
Code: Select all
int variable = 0; // initialize your variable
Convert.ToInt32(TextBox1.Text); // textbox.text returns a string, so convert it to an int
Code: Select all
int num1; // For storing the integer (whole number)
if (int.TryParse(textBox1.Text, out num1))
{
// If we're here that means the text could be converted to an integer, and so num1 will now be that variable integer
}
else
{
// Couldn't convert
}
Halo 2 Prophet - Skin with ease with the simple 3D point and click interface.
Halo 3 Research Thread - Contribute to the research into Halo 3.
Halo 3 Research Thread - Contribute to the research into Halo 3.
- WaywornMmmmm
- Posts: 1341
- Joined: Sat Nov 05, 2005 5:17 am
- Location: U.S.A
![]() |
I tried your's prey, but I get an error saying int doesn't contain a deffinition for TryParse.
I also tried OwnZ joO's but I don't really understand it.
Remeber that I am very new to this so explanation in great detail will help.
Edit: After searching google for a while, I found a solution that works for me.
Now I have a new question. Is it possible to make the datatype of a variable that is a square root not a double?
Basicly, can I get somthing like this
I also tried OwnZ joO's but I don't really understand it.
Remeber that I am very new to this so explanation in great detail will help.
Edit: After searching google for a while, I found a solution that works for me.
Now I have a new question. Is it possible to make the datatype of a variable that is a square root not a double?
Basicly, can I get somthing like this
Code: Select all
int variable = (Math.Sqrt(otherVariable));
Code: Select all
int variable = (Math.Sqrt(otherVariable));
Code: Select all
int variable = (int)Math.Sqrt(yourDouble);
- WaywornMmmmm
- Posts: 1341
- Joined: Sat Nov 05, 2005 5:17 am
- Location: U.S.A
![]() |

Me wonders what C# you are using :/
Halo 2 Prophet - Skin with ease with the simple 3D point and click interface.
Halo 3 Research Thread - Contribute to the research into Halo 3.
Halo 3 Research Thread - Contribute to the research into Halo 3.
- LuxuriousMeat
- Posts: 824
- Joined: Thu Nov 03, 2005 6:43 pm
- Location: zzzzzzzzzzzzzzzz
- Contact:
If you can't get TryParse to work do this:
Code: Select all
int num = 0;
try
{
// parse the int
num = int.Parse(textBox1.Text);
}
catch
{
// if it reaches here the text cant be parsed to an int
}
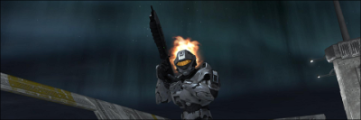
- WaywornMmmmm
- Posts: 1341
- Joined: Sat Nov 05, 2005 5:17 am
- Location: U.S.A
![]() |
Re: Some C# Questions
Not sure what your asking here, but the 'Math.' does have quite a few useful functions which may be what your looking for.WaywornMmmmm wrote:Is there a way to have c# to check if a variable is a perfect square?
You can make a form a MDIContainer by enabing the 'IsMdiContainer' in the forms properties, then to add a form to the container:WaywornMmmmm wrote:Also, is there a way to add a new form to my project which will be an MDIContainer for the original and any new forms I may add?
Code: Select all
private void Form1_Load(object sender, EventArgs e)
{
Form2 form2 = new Form2();
form2.MdiParent = this;
form2.Show();
}
Halo 2 Prophet - Skin with ease with the simple 3D point and click interface.
Halo 3 Research Thread - Contribute to the research into Halo 3.
Halo 3 Research Thread - Contribute to the research into Halo 3.
- WaywornMmmmm
- Posts: 1341
- Joined: Sat Nov 05, 2005 5:17 am
- Location: U.S.A
![]() |
Re: Some C# Questions
What I am trying to do is have C# check to see if my variable is a perfect square (4,9,16,25,36, etc.) and if not display a message box with that number. I know how to do the latter, but I am not sure how to do the first part.Prey wrote:Not sure what your asking here, but the 'Math.' does have quite a few useful functions which may be what your looking for.WaywornMmmmm wrote:Is there a way to have c# to check if a variable is a perfect square?
Also, when I try to make the form I added to my project an MDIContainer with your code, I get an error saying that my form can't be both and MDIParent and an MDIContainer
What I did was add my form, set its Is MdiContainer property to true, then went the the load event of my original form and entered in the code you gave me.

For your square root problem here's a possible solution.(note: its sloppy and there's probably better ways to do this but it works...)
Code: Select all
double d = # you want to check;
long i = (long)Math.Sqrt(d); // you want to convert the sqrt to a value that does not hold decimals. ie: integers, longs, etc...
if ((i * i) == d)
{
// it's a square #
}
else
{
// it's not a square #
}
Re: Some C# Questions
For a MdiContainer
1) Go to the properties of the form you wish to be a container.
2) Enable 'IsMdiContainer'.
3) Now go into the containers load event. Assuming the container form is 'Form1' and the form you want to add is 'Form2'; the code i posted above will work just fine.
As for finding out if the number is a perfect square, dos mes' way would work, but here is a faster alternative:
1) Go to the properties of the form you wish to be a container.
2) Enable 'IsMdiContainer'.
3) Now go into the containers load event. Assuming the container form is 'Form1' and the form you want to add is 'Form2'; the code i posted above will work just fine.
As for finding out if the number is a perfect square, dos mes' way would work, but here is a faster alternative:
Code: Select all
double d = 13; // The squared num
double sqrt = Math.Sqrt(d); // The square root of the num
if (sqrt == Math.Round(sqrt))
{
// No rounding was done, thus the number is a whole
}
else
{
// Rounding was done, thus the number wasn't a whole
}
Halo 2 Prophet - Skin with ease with the simple 3D point and click interface.
Halo 3 Research Thread - Contribute to the research into Halo 3.
Halo 3 Research Thread - Contribute to the research into Halo 3.
- WaywornMmmmm
- Posts: 1341
- Joined: Sat Nov 05, 2005 5:17 am
- Location: U.S.A
![]() |
When I input your code into the load event for the MdiContainer, with the form names and coding exactly the same:
Form2 loads when I test it, but Form1 doesn't. I've used this code once before when the MdiContainer was the first form in my app, and it worked. I don't think this code works when the MdiContainer is added to the app.
Code: Select all
Form2 form2 = new Form2();
form2.MdiParent = this;
form2.Show();
So what are you trying to say? You trying to add Form1 to the MdiContainer too?
Remember that the 'this' is the current form, and so the current form must be the MdiContainer
Code: Select all
Form1 form1 = new Form1();
form1.MdiParent = this;
form1.Show();
Halo 2 Prophet - Skin with ease with the simple 3D point and click interface.
Halo 3 Research Thread - Contribute to the research into Halo 3.
Halo 3 Research Thread - Contribute to the research into Halo 3.