Page 1 of 1
Even and Odd
Posted: Fri Jul 20, 2007 11:47 pm
by Patrickh
Still using VB.
sorry C# guys
dad got me a book
so i made a random number generator where you put down 2 values in text boxes and it generates a number between them (when you hit cmdGenerate)
then I wanted to add options for evens only and odds only, but I don't know how. here's what I have, I just need to know how to make it so the generated # is even for the first case, and odd for the second case
Code: Select all
Private Sub cmdGenerate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdGenerate.Click
If IsNumeric(TextBox1.Text) And IsNumeric(TextBox2.Text) Then
Select Case True
Case optEven.Checked
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text)
Case optOdd.Checked
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text)
Case Else
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text)
End Select
Else
MsgBox("Not a number, idiot.", MsgBoxStyle.Exclamation, "Error")
End If
End Sub
thanks
Posted: Sat Jul 21, 2007 12:20 am
by xbox7887
For an even case, do a logical AND of the number by 0xFFFFFFFE and for an odd case do a logical OR by 1.
Posted: Sat Jul 21, 2007 9:52 am
by Patrickh
xbox7887 wrote:For an even case, do a logical AND of the number by 0xFFFFFFFE and for an odd case do a logical OR by 1.
thanks for helping me, but can you be a bit more specific? I'm new to programming. Are you saying I need to do an And/Or statement?
Posted: Sat Jul 21, 2007 10:27 am
by xbox7887
I've never used visual basic before but I believe they spell out their stuff so I would try something along the lines of...
Code: Select all
int number = Random().Next();
int evenNumber = number AND 0xFFFFFFFE;
int oddNumber = number OR 1;
Also when referencing hexidecimal, they may use a different format like 0FFFFFFFEH...you should be able to figure it out from there. And pay no attention to my C-style variable declarations, use the Dim bullshit that VB makes you do

Posted: Sat Jul 21, 2007 12:39 pm
by LuxuriousMeat
Use this:
Code: Select all
Private Sub cmdGenerate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdGenerate.Click
If IsNumeric(TextBox1.Text) And IsNumeric(TextBox2.Text) Then
Select Case True
Case optEven.Checked
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text) And Integer.Parse("FFFFFFFE", Globalization.NumberStyles.HexNumber)
Case optOdd.Checked
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text) Or 1
Case Else
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text)
End Select
Else
MsgBox("Not a number, idiot.", MsgBoxStyle.Exclamation, "Error")
End If
End Sub
Posted: Sat Jul 21, 2007 12:54 pm
by Patrickh
LuxuriousMeat wrote:Use this:
Code: Select all
Private Sub cmdGenerate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdGenerate.Click
If IsNumeric(TextBox1.Text) And IsNumeric(TextBox2.Text) Then
Select Case True
Case optEven.Checked
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text) And Integer.Parse("FFFFFFFE", Globalization.NumberStyles.HexNumber)
Case optOdd.Checked
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text) Or 1
Case Else
Dim objRandom As System.Random
objRandom = New Random(Now.Millisecond)
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text)
End Select
Else
MsgBox("Not a number, idiot.", MsgBoxStyle.Exclamation, "Error")
End If
End Sub
that worked, thanks!
Posted: Sat Jul 21, 2007 4:00 pm
by Prey
Seeing as the 'Random' class is always used you might as well declare that at the start, and you might as well make the hex conversion more "vb"-ish too.
Code: Select all
If IsNumeric(TextBox1.Text) And IsNumeric(TextBox2.Text) Then
Dim objRandom = New Random()
Select Case True
Case optEven.Checked
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text) And Val("&HFFFFFFFE")
Case optOdd.Checked
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text) Or 1
Case Else
lblRandomNumber.Text = objRandom.Next(TextBox1.Text, TextBox2.Text)
End Select
Else
MsgBox("Not a number, idiot.", MsgBoxStyle.Exclamation, "Error")
End If
Posted: Sat Jul 21, 2007 11:26 pm
by Patrickh
Thanks prey. One small problem, I was playing with the program and I entered 3 and 5, then checked the evens box. It spit out 4s and
2s
does anyone know why this is? or possible solutions?
and yes, it does it every time, I closed the app and tried again with the same results
(yes the evens box is checked, but it's under more options
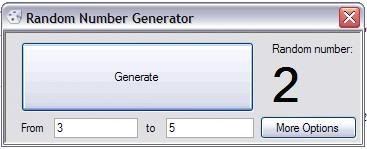
Posted: Sun Jul 22, 2007 8:27 am
by xbox7887
Because you're still running your even odd code...if it randomly generates a 3 (which is your min), then the even code will lop off the ones bit and make it a two...
Basically, you get a random number...if you want it odd the code rounds up, if you want it even the code rounds down ;p
Posted: Sun Jul 22, 2007 10:56 am
by Prey
You could put in a few while statements then that'll keep looping until the number is within its boundaries,
Code: Select all
If IsNumeric(TextBox1.Text) And IsNumeric(TextBox2.Text) Then
'first we run a few tests to make sure there is at least a gap of two
Dim varDiff As Integer = TextBox2.Text - TextBox1.Text
If varDiff = 0 Or varDiff = 1 Or varDiff = -1 Then End
'If we're still here then yes there is an 'in-between'. Without those
'tests the while loops could have potentially looped on forever
'Declare
Dim objRandom = New Random()
Dim varRandom As Integer = TextBox1.Text - 1
'Get random integer
Select Case True
Case optEven.Checked
'If the var is smaller or equal to textbox1's text, or the var
'is bigger than or equal to textbox2's text; continue looping
While varRandom <= TextBox1.Text Or varRandom >= TextBox2.Text
varRandom = objRandom.Next(TextBox1.Text, TextBox2.Text) And Val("&HFFFFFFFE")
End While
Case optOdd.Checked
While varRandom <= TextBox1.Text Or varRandom >= TextBox2.Text
varRandom = objRandom.Next(TextBox1.Text, TextBox2.Text) Or 1
End While
Case Else
varRandom = objRandom.Next(TextBox1.Text, TextBox2.Text)
End Select
'Display
lblRandomNumber.Text = varRandom
Else
'Error handling
lblRandomNumber.Text = "Not a number, idiot."
End If
Posted: Sun Jul 22, 2007 12:04 pm
by OwnZ joO
Another way to determine if a number is even or odd is to divide it by 2 and if the remainder is 0 then it's even
I'm really not sure on my vb.net code anymore, but i think it would look something like this just for determining if it's even:
Code: Select all
Private Function IsEven(byval number As Integer)As Boolean
int rem = number mod 2 ' mod returns the remainder of division
return rem = 0 ' if it's remainder is 0 return true, else false
End Function
Posted: Sun Jul 22, 2007 7:47 pm
by Patrickh
Wow, you guys are really helpful!
Here is the solution I came up with, it fixes the problem fine, but let me know if this is bad practice or something. All I did was add this:
Code: Select all
If CInt(lblRandomNumber.Text) < CInt(TextBox1.Text) Then
cmdGenerate.PerformClick()
End If
That way if the number isn't equal to or above the min value, it tries again, and so on, until it generates a proper number
Posted: Mon Jul 23, 2007 2:26 am
by Prey
Patrickh wrote:Wow, you guys are really helpful!
Here is the solution I came up with, it fixes the problem fine, but let me know if this is bad practice or something. All I did was add this:
Code: Select all
If CInt(lblRandomNumber.Text) < CInt(TextBox1.Text) Then
cmdGenerate.PerformClick()
End If
That way if the number isn't equal to or above the min value, it tries again, and so on, until it generates a proper number
Yea ok, but that doesn't take into account if the number is bigger than TextBox2. Also you shouldn't have to 'CInt', vB should take care of that for you. One last thing, is that code in the 'cmdGenerate.PerformClick()' method? Because otherwise the number returned could again be outside the boundaries and you wouldn't have any to code left to check again.
Posted: Mon Jul 23, 2007 11:27 am
by Patrickh
Prey wrote:Patrickh wrote:Wow, you guys are really helpful!
Here is the solution I came up with, it fixes the problem fine, but let me know if this is bad practice or something. All I did was add this:
Code: Select all
If CInt(lblRandomNumber.Text) < CInt(TextBox1.Text) Then
cmdGenerate.PerformClick()
End If
That way if the number isn't equal to or above the min value, it tries again, and so on, until it generates a proper number
Yea ok, but that doesn't take into account if the number is bigger than TextBox2. Also you shouldn't have to 'CInt', vB should take care of that for you. One last thing, is that code in the 'cmdGenerate.PerformClick()' method? Because otherwise the number returned could again be outside the boundaries and you wouldn't have any to code left to check again.
Yes it is the last thing inside the click event, so if the number is less than the min it just tries again. as for the greater than textbox2, now that i think about it i should add that, because if you asked for an odd number between 2 and 4 it would give you five. good call
